for insecure uses, generate a “special” password here: https://myaccount.google.com/u/2/apppasswords?rapt=AEjHLxxxxxx
“special” password is used for mailx (mail.rc) and SMTPMailer.php and insecure nodeMailer
mailx
mailx – uses /etc/mail.rc uses password generated in the step above.
EXAMPLE:
echo `date` | /usr/bin/mailx -A gmailSMTP-noreply -s “test subject TEST” mark@edwardsmark.com ;
account gmailSMTP-noreply { set smtp-use-starttls set ssl-verify=ignore set smtp-auth=login set smtp=smtp://smtp.gmail.com:587 set smtp-auth-user=noreply@comptonpeslonline.com set smtp-auth-password=gXXXXv set ssl-verify=ignore set nss-config-dir=/home/comptonpeslonline.com/gmailCerts/ }
php
SMTPMailer.php:define (‘DEFAULT_EMAIL’ , ‘noreply@comptonpeslonline.com’ ); define (‘PORT25TEST’ , ‘check-auth-edwardsmarkf=gmail.com@verifier.port25.com’ ); define (‘DEFAULT_SMTP_HOST’ , ‘smtp.gmail.com’ ); // 2016-12-05 define (‘DEFAULT_SMTP_LOGIN’ , ‘noreply@comptonpeslonline.com’ ); // 2016-12-05 define (‘DEFAULT_SMTP_PASSWD’ , ‘gXXXXXXXv’ );
nodeMailer Insecure
const nodemailer = require('nodemailer') ; const authEmailAddy = 'info@comptonpeslonline.com' ; const authPassword = 'zmcafzfppvquyqjj' ; const transporter = nodemailer.createTransport( { service : 'gmail' , auth : { user : authEmailAddy , pass : authPassword } } ); const mailOptions = { from : authEmailAddy , to : 'mark@edwardsmark.com' //, cc : teacherNameAndEmail , bcc : 'mark@edwardsmark.com' , subject : 'test subject from testSecureMailer.js' , text : 'test text body from testSecureMailer.js' , html : '<h3>test html body from testSecureMailer.js</h3>' }; transporter.sendMail(mailOptions, (err, res) => { if (err) { console.log('Failed mailing to ' + ': ' + JSON.stringify(res)); return console.log(err); } else { console.log('Successful mailing to ' + userObject.userEmail + ': ' + JSON.stringify(res)); } });
nodeMailer with Oauth
node uses an “auth0” approach:
step one:
log out of all accounts (or do all this in firefox)step two:
https://console.cloud.google.com/apis/credentials/oauthclient/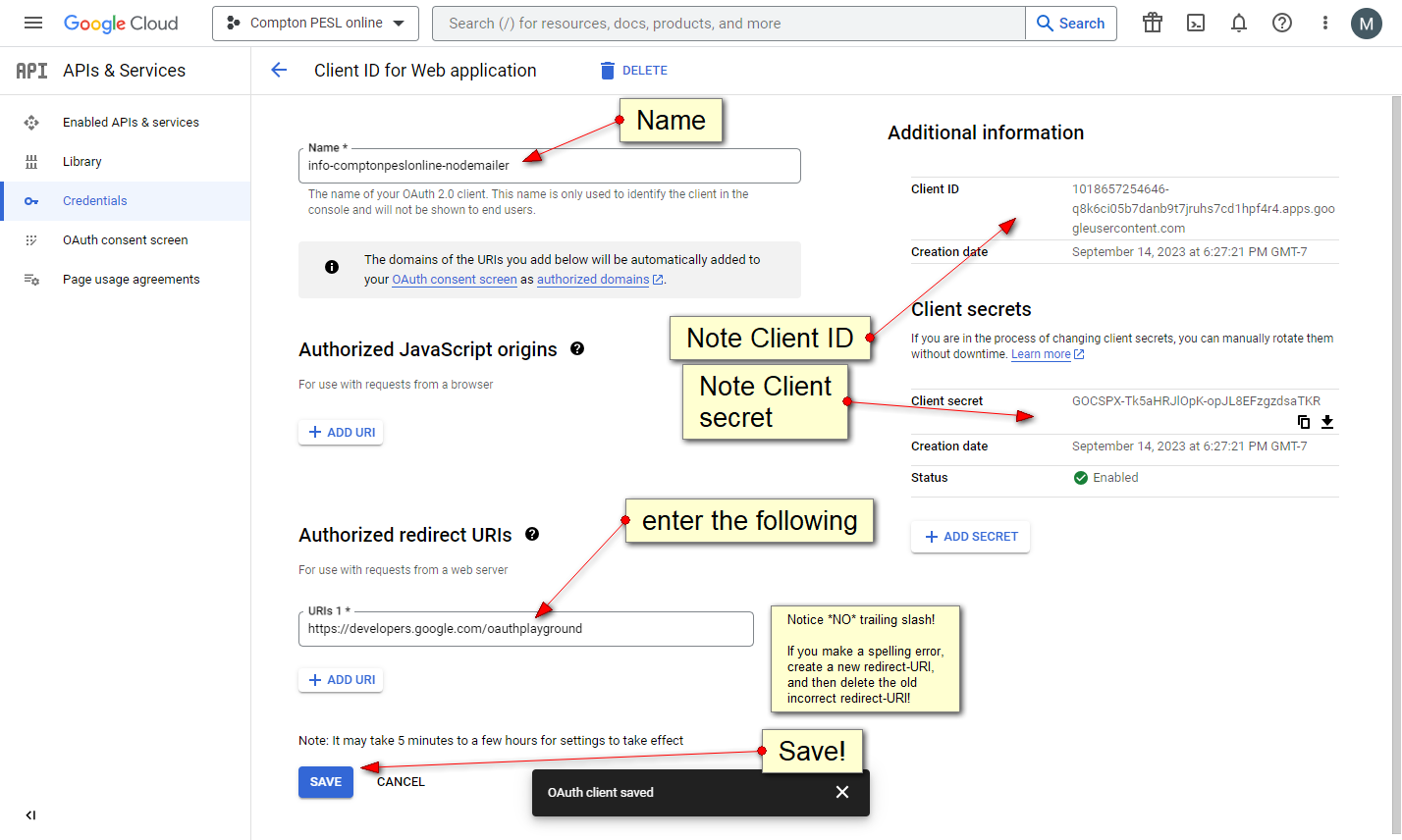
step three:
enter https://developers.google.com/oauthplayground/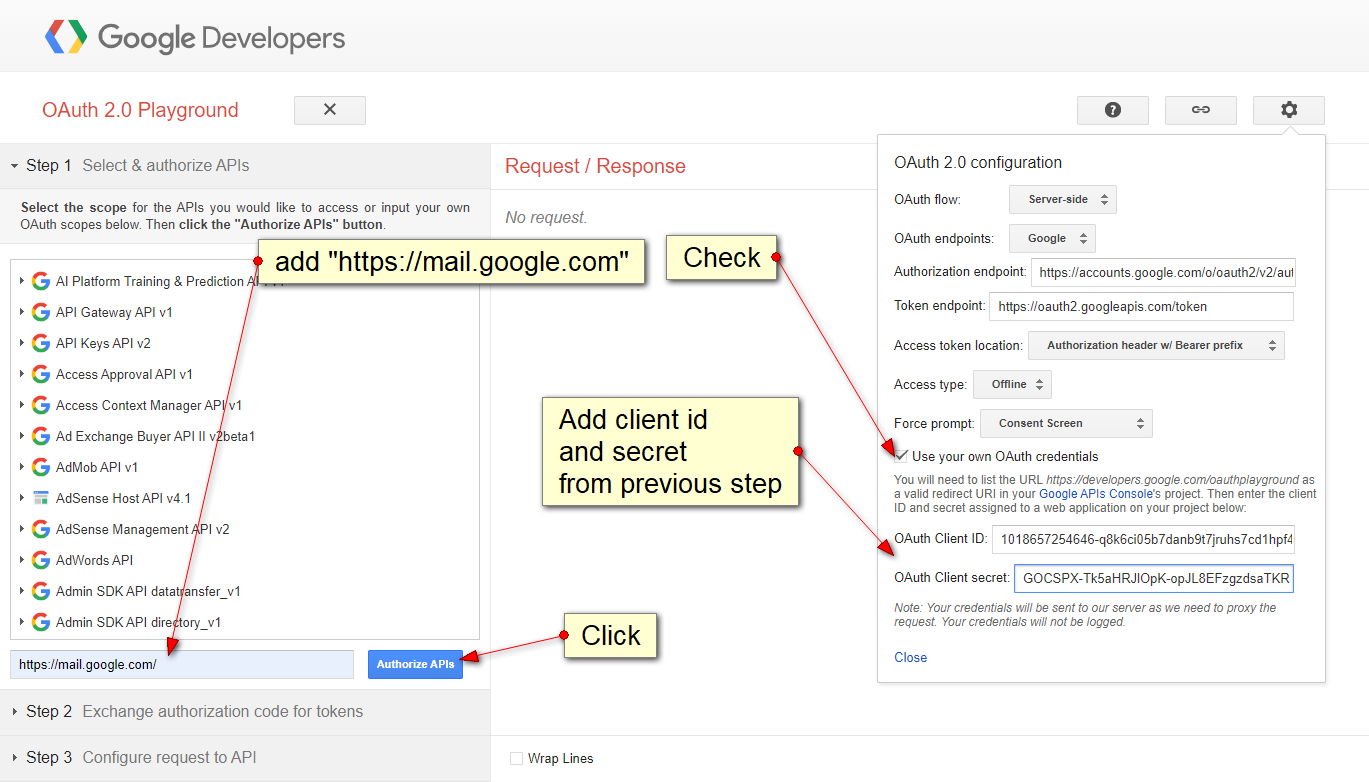
step four:
approve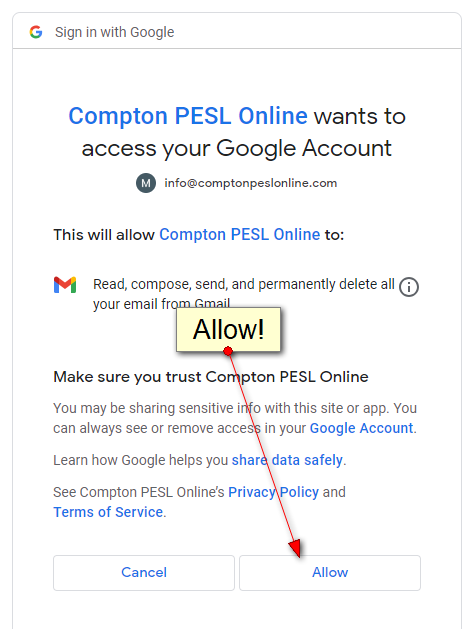
step five:
create and copy the Refresh token: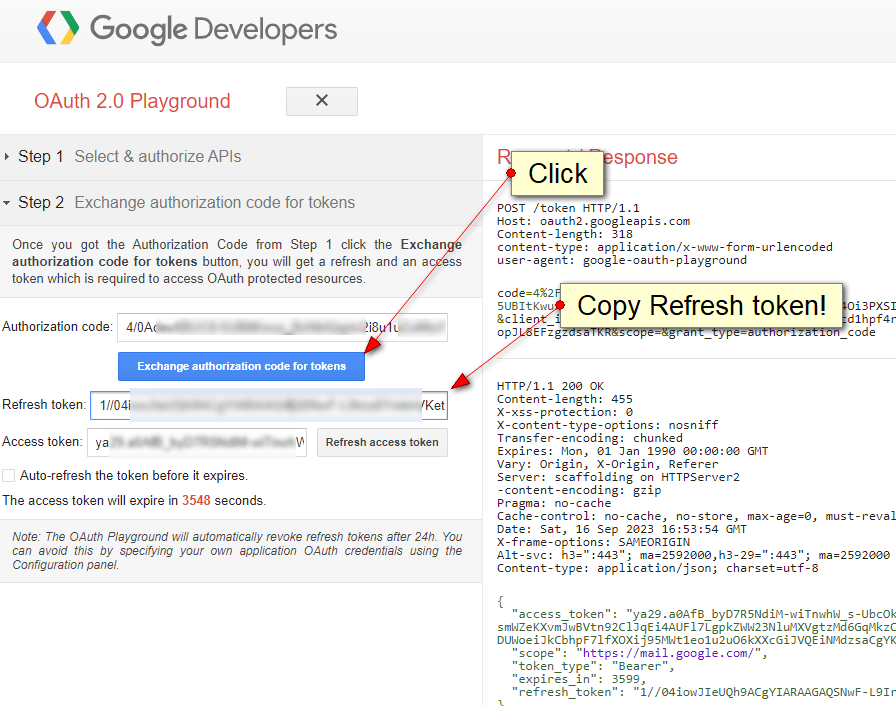
const nodemailer = require(‘nodemailer’) ; const authEmailAddy = ‘noreply@comptonpeslonline.com’ ; const clientId = ‘6884XXXXXXh6n.apps.googleusercontent.com’ ; const clientSecret = ‘SXXXXXXS’ ; const refreshToken = ’1//0XXXXCq4’ ; const auth = { type : ‘oauth2’ , user : authEmailAddy , clientId , clientSecret , refreshToken }; const transporter = nodemailer.createTransport( { service : ‘gmail’ , auth } );
node example: /home/comptonpeslonline.com/public_html/comptonPractice/homeworkAssignment/ticklerMailer/ticklerMailer.js
written from:
https://tanaikech.github.io/2018/01/08/send-mails-from-gmail-using-nodemailer/
https://medium.com/@nickroach_50526/sending-emails-with-node-js-using-smtp-gmail-and-oauth2-316fe9c790a1
https://dev.to/documatic/send-email-in-nodejs-with-nodemailer-using-gmail-account-2gd1
https://stackoverflow.com/questions/24098461/nodemailer-gmail-what-exactly-is-a-refresh-token-and-how-do-i-get-one
https://stackoverflow.com/questions/72128433/authenticating-google-gmail-api-using-access-and-refresh-token
2023-09-14 ================================================
to bypass creating an account in /etc/mail.rc :
1) add new password in /etc/postfix/sasl/sasl_passwd
2) postmap -v /etc/postfix/sasl/sasl_passwd ;
3) systemctl reload postfix ; systemctl restart postfix ; systemctl status postfix ;